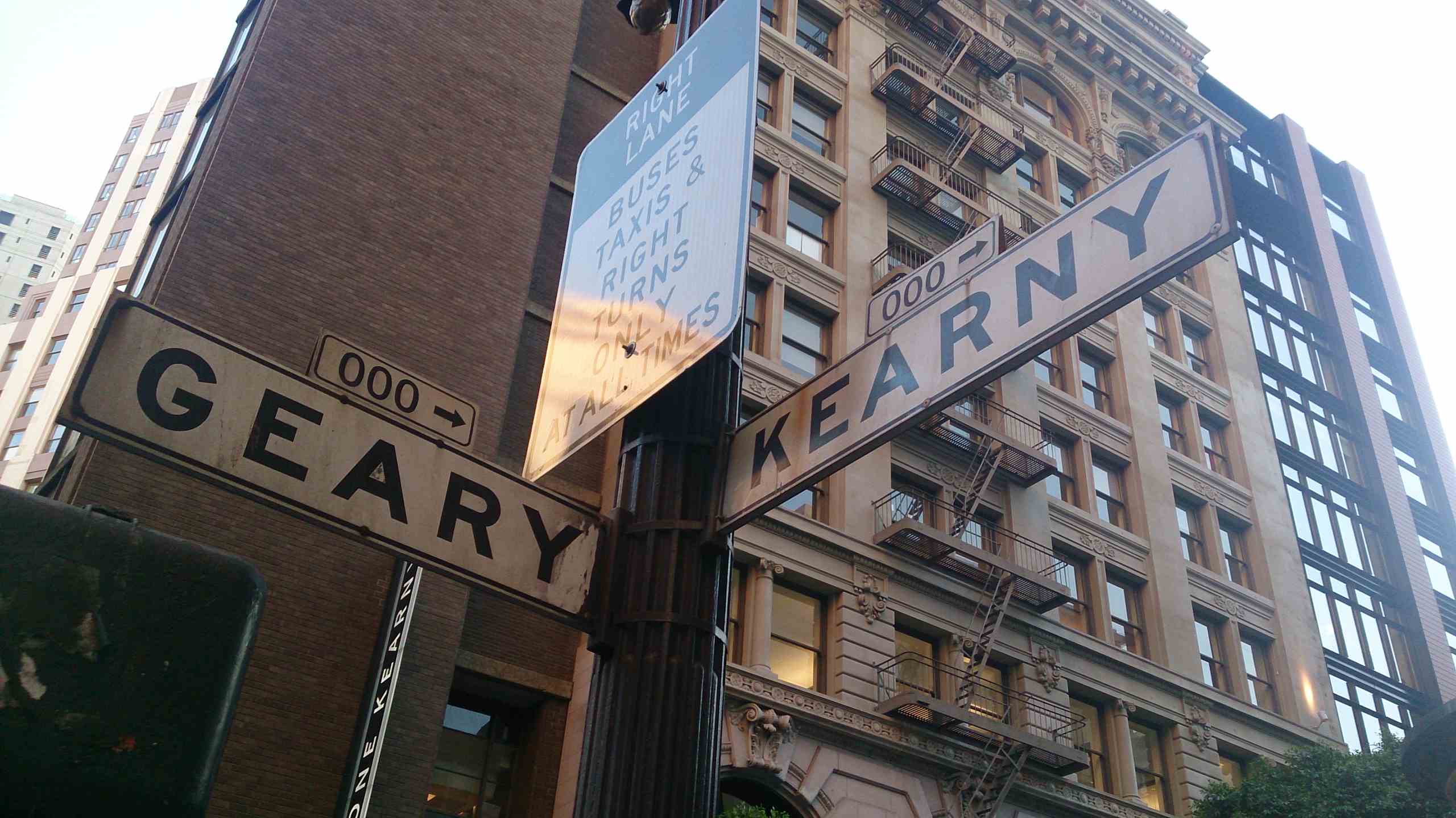
Ruby on Raylsで複数DBを使いたいメモ
コツ
* 接続情報をネストする
* migrations_paths
でmigrationファイルの場所を分ける
* 接続だけしたい(migrationとか不要)ならdatabase_tasks: false
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
development:
main:
<<: *default
database: multi_db_db_main_development
migrations_paths: db/main
host: db_main
sub:
<<: *default
database: multi_db_db_sub_development
migrations_paths: db/sub
host: db_sub
# database_tasks: false
test:
main:
<<: *default
database: multi_db_db_main_test
migrations_paths: db/main
host: db_main
sub:
<<: *default
database: multi_db_db_sub_test
migrations_paths: db/sub
host: db_sub
production
...
--database
オプションを付与する
接続先を指定したApplicationRecordのサブクラスが一緒に作られる(なければ)
各モデルはApplicationRecordの孫クラスになる
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
$ bin/rails g model dog name:string --database main
invoke active_record
create db/main/20230209193743_create_dogs.rb
create app/models/main_record.rb
create app/models/dog.rb
$ bin/rails g model cat name:string --database sub
invoke active_record
create db/sub/20230209194008_create_cats.rb
create app/models/sub_record.rb
create app/models/cat.rb
$ bin/rails g model tiger name:string --database sub
invoke active_record
create db/sub/20230209194036_create_tigers.rb
create app/models/tiger.rb
例えばsubが管理外の既存データベースで、いきなりモデルを作成するような場合はMainRecordとSubRecordを手動で作成する必要がある。
main
1
2
3
4
5
class MainRecord < ApplicationRecord
self.abstract_class = true
connects_to database: { writing: :main }
end
sub
1
2
3
4
5
6
class SubRecord < ApplicationRecord
self.abstract_class = true
connects_to database: { writing: :sub }
after_initialize :readonly! # dbへの書き込みを禁止したい場合
end
connects_to には readingオプションも渡せる。
リードレプリカに接続したいときに使う。
1
2
3
4
5
$ rails db:create
Created database 'multi_db_db_main_development'
Created database 'multi_db_db_sub_development'
Created database 'multi_db_db_main_test'
Created database 'multi_db_db_sub_test'
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
$ rails db:migrate
== 20230209193743 CreateDogs: migrating =======================================
-- create_table(:dogs)
-> 0.0242s
== 20230209193743 CreateDogs: migrated (0.0243s) ==============================
== 20230209194008 CreateCats: migrating =======================================
-- create_table(:cats)
-> 0.0232s
== 20230209194008 CreateCats: migrated (0.0233s) ==============================
== 20230209194036 CreateTigers: migrating =====================================
-- create_table(:tigers)
-> 0.0250s
== 20230209194036 CreateTigers: migrated (0.0251s) ============================
1
2
3
4
5
6
7
8
9
10
irb(main):001:0> Dog.create(name: '柴犬')
TRANSACTION (0.2ms) BEGIN
Dog Create (0.4ms) INSERT INTO `dogs` (`name`, `created_at`, `updated_at`) VALUES ('柴犬', '2023-02-09 19:42:27.364216', '2023-02-09 19:42:27.364216')
TRANSACTION (5.0ms) COMMIT
=> #<Dog:0x00007ffa2c8290f0 id: 1, name: "柴犬", created_at: Fri, 10 Feb 2023 04:42:27.364216000 JST +09:00, updated_at: Fri, 10 Feb 2023 04:42:27.364216000 JST +09:00>
irb(main):002:0> Cat.create(name: 'マンチカン')
TRANSACTION (0.2ms) BEGIN
Cat Create (0.3ms) INSERT INTO `cats` (`name`, `created_at`, `updated_at`) VALUES ('マンチカン', '2023-02-09 19:42:31.738822', '2023-02-09 19:42:31.738822')
TRANSACTION (4.3ms) COMMIT
=> #<Cat:0x00007ffa2c73e118 id: 1, name: "マンチカン", created_at: Fri, 10 Feb 2023 04:42:31.738822000 JST +09:00, updated_at: Fri, 10 Feb 2023 04:42:31.738822000 JST +09:00>
main
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
MySQL [multi_db_db_main_development]> show databases;
+------------------------------+
| Database |
+------------------------------+
| information_schema |
| multi_db_db_main_development |
| multi_db_db_main_test |
| multi_db_main_development |
| mysql |
| performance_schema |
| sys |
+------------------------------+
7 rows in set (0.001 sec)
MySQL [multi_db_db_main_development]> show tables;
+----------------------------------------+
| Tables_in_multi_db_db_main_development |
+----------------------------------------+
| ar_internal_metadata |
| dogs |
| schema_migrations |
+----------------------------------------+
3 rows in set (0.001 sec)
MySQL [multi_db_db_main_development]> select * from dogs;
+----+--------+----------------------------+----------------------------+
| id | name | created_at | updated_at |
+----+--------+----------------------------+----------------------------+
| 1 | 柴犬 | 2023-02-09 19:42:27.364216 | 2023-02-09 19:42:27.364216 |
+----+--------+----------------------------+----------------------------+
sub
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
MySQL [multi_db_db_sub_development]> show databases;
+-----------------------------+
| Database |
+-----------------------------+
| information_schema |
| multi_db_db_sub_development |
| multi_db_db_sub_test |
| multi_db_sub_development |
| mysql |
| performance_schema |
| sys |
+-----------------------------+
7 rows in set (0.001 sec)
MySQL [multi_db_db_sub_development]> show tables;
+---------------------------------------+
| Tables_in_multi_db_db_sub_development |
+---------------------------------------+
| ar_internal_metadata |
| cats |
| schema_migrations |
| tigers |
+---------------------------------------+
4 rows in set (0.001 sec)
MySQL [multi_db_db_sub_development]> select * from cats;
+----+-----------------+----------------------------+----------------------------+
| id | name | created_at | updated_at |
+----+-----------------+----------------------------+----------------------------+
| 1 | マンチカン | 2023-02-09 19:42:31.738822 | 2023-02-09 19:42:31.738822 |
+----+-----------------+----------------------------+----------------------------+
mainにはmainのsubにはsubのテーブルができて、それぞれにデータ登録できた。
めでたし
コメント